Learn how to evaluate and integrate the VNC SDK
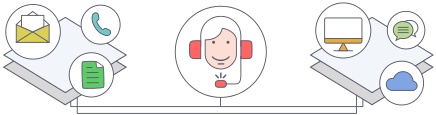
Viewer¶
-
vncsdk.
Viewer
¶ A VNC-compatible Viewer showing the screen of and controlling a remote computer.
Nested Classes¶
- class
vncsdk.Viewer.ViewerCanvasContext()
vncsdk.Viewer.ConnectionStatus
vncsdk.Viewer.DisconnectFlags
vncsdk.Viewer.EncryptionLevel
vncsdk.Viewer.MouseButton
vncsdk.Viewer.MouseWheel
vncsdk.Viewer.PictureQuality
vncsdk.Viewer.AuthenticationCallback
vncsdk.Viewer.ConnectionCallback
vncsdk.Viewer.FramebufferCallback
vncsdk.Viewer.PeerVerificationCallback
vncsdk.Viewer.ServerEventCallback
vncsdk.Viewer.ServerPointerCallback
Constructor Summary¶
Modifier and Type | Method and Description |
---|---|
Viewer() Creates and returns a new viewer. |
Method Summary¶
Modifier and Type | Method and Description |
---|---|
Uint8Array or null |
Returns the viewer framebuffer data for the given rectangle. |
A painting function that paints the framebuffer into a canvas, using the most optimised functions that the browser provides. |
|
Destroys the viewer. |
|
Disconnects this viewer from the server. |
|
Enables receipt of audio from the Server by the Viewer. |
|
vncsdk.AnnotationManager |
Obtains the Viewer’s |
vncsdk.ConnectionHandler |
Returns the viewer’s |
vncsdk.Viewer.ConnectionStatus |
Returns the status of the viewer’s connection. |
string or null |
Returns a human-readable message sent by the server for the last disconnection, or |
string or null |
Returns a string ID representing the reason for the last viewer disconnection. |
vncsdk.DisplayManager |
Obtains the Viewer’s |
vncsdk.Viewer.EncryptionLevel |
Returns the Viewer’s current encryption level. |
vncsdk.MessagingManager |
Obtains the Viewer’s Messaging Manager for handling messaging For more information, see |
string |
Returns the address of the viewer’s server. |
vncsdk.Viewer.PictureQuality |
Returns the viewer’s current picture quality. |
vncsdk.DataBuffer |
Gets the current mouse cursor image from the Server for rendering locally. |
number |
Gets the height of the pointer framebuffer. |
vncsdk.PixelFormat |
Gets the pixel format of the pointer framebuffer. |
number |
Gets the width of the pointer framebuffer. |
number |
Gets the X offset of the “hotspot” of the pointer. |
number |
Gets the Y offset of the “hotspot” of the pointer. |
number |
Gets the height of the viewer framebuffer. |
vncsdk.PixelFormat |
Gets the pixel format of the viewer framebuffer. |
number |
Returns the stride of the viewer framebuffer data in pixels, that is, the number of pixels from the start of each row until the start of the next. |
number |
Gets the width of the viewer framebuffer. |
Send key up events for all currently pressed keys. |
|
Provides the SDK with the result of a username/password request. |
|
Copies the given text to the server’s clipboard. |
|
Sends a key down (press) event to the server. |
|
Sends a key up (release) event to the server. |
|
Provides the SDK with the response to the |
|
Sends a pointer event to the server. |
|
Sends a scroll wheel event to the server. |
|
Sets the callback to be called when a username and/or password is required. |
|
Sets the callbacks for the Viewer to call when various events occur during its lifetime. |
|
Sets the desired encryption level of the session from the range of options enumerated by :js:class:`vncsdk.Viewer`_EncryptionLevel. |
|
Sets the framebuffer callback for this viewer. |
|
Sets the callbacks to be called to verify the identity of the peer (server). |
|
Sets the desired picture quality of the session from the range of options enumerated by :js:class:`vncsdk.Viewer`_PictureQuality. |
|
Sets the server event callback for this viewer. |
|
Sets the server pointer callback for this viewer. |
|
Sets the pixel format of the pointer framebuffer. |
|
Sets the rate with which serverPointerPos callback will be called. |
|
Sets the viewer framebuffer. |
Constructor¶
-
class
vncsdk.
Viewer
() Creates and returns a new viewer.
For more information, see
vnc_Viewer_create()
.Throws: vncsdk.VncException
on error
Methods¶
-
Viewer.
getViewerFbData
(x, y, w, h)¶ Returns the viewer framebuffer data for the given rectangle. This method is rarely useful, and is inefficient. Typically, efficient access to the framebuffer is provided by using
Viewer.putViewerFbData()
to paint directly into an HTML canvas.Arguments: - x (number) –
- y (number) –
- w (number) –
- h (number) –
Throws: vncsdk.VncException
on errorReturn type: Uint8Array or null
-
Viewer.
putViewerFbData
(x, y, w, h, canvasCtx[, targetX[, targetY]])¶ A painting function that paints the framebuffer into a canvas, using the most optimised functions that the browser provides. It uses a helper class
vncsdk.Viewer.ViewerCanvasContext
which stores the canvas and buffer context in between calls. TheViewerCanvasContext
should be created once and passed in to each successive call toputViewerFbData()
. IftargetX
andtargetY
are not provided, they default to 0. The viewer’s pixel format must be set to RGBX (ie. little-endianvncsdk.PixelFormat.bgr888
).Arguments: - x (number) –
- y (number) –
- w (number) –
- h (number) –
- canvasCtx (
vncsdk.Viewer.ViewerCanvasContext
) – - targetX (number) – Defaults to 0
- targetY (number) – Defaults to 0
Throws: vncsdk.VncException
on error
-
Viewer.
destroy
()¶ Destroys the viewer.
For more information, see
vnc_Viewer_destroy()
.
-
Viewer.
disconnect
()¶ Disconnects this viewer from the server.
For more information, see
vnc_Viewer_disconnect()
.Throws: vncsdk.VncException
on error
-
Viewer.
enableAudio
(enable)¶ Enables receipt of audio from the Server by the Viewer.
For more information, see
vnc_Viewer_enableAudio()
.Arguments: - enable (boolean) –
Throws: vncsdk.VncException
on error
-
Viewer.
getAnnotationManager
()¶ Obtains the Viewer’s
vncsdk.AnnotationManager()
for handling annotation operations.For more information, see
vnc_Viewer_getAnnotationManager()
.Return type: vncsdk.AnnotationManager
-
Viewer.
getConnectionHandler
()¶ Returns the viewer’s
vncsdk.ConnectionHandler()
for accepting connections.For more information, see
vnc_Viewer_getConnectionHandler()
.Return type: vncsdk.ConnectionHandler
-
Viewer.
getConnectionStatus
()¶ Returns the status of the viewer’s connection.
For more information, see
vnc_Viewer_getConnectionStatus()
.Return type: vncsdk.Viewer.ConnectionStatus
-
Viewer.
getDisconnectMessage
()¶ Returns a human-readable message sent by the server for the last disconnection, or
null
if the last disconnection was not initiated by the server.For more information, see
vnc_Viewer_getDisconnectMessage()
.Return type: string or null
-
Viewer.
getDisconnectReason
()¶ Returns a string ID representing the reason for the last viewer disconnection.
For more information, see
vnc_Viewer_getDisconnectReason()
.Return type: string or null
-
Viewer.
getDisplayManager
()¶ Obtains the Viewer’s
vncsdk.DisplayManager()
, for managing the list of displays made available by the Server to the Viewer.For more information, see
vnc_Viewer_getDisplayManager()
.Return type: vncsdk.DisplayManager
-
Viewer.
getEncryptionLevel
()¶ Returns the Viewer’s current encryption level.
For more information, see
vnc_Viewer_getEncryptionLevel()
.Return type: vncsdk.Viewer.EncryptionLevel
-
Viewer.
getMessagingManager
()¶ Obtains the Viewer’s Messaging Manager for handling messaging
For more information, see
vnc_Viewer_getMessagingManager()
.Return type: vncsdk.MessagingManager
-
Viewer.
getPeerAddress
()¶ Returns the address of the viewer’s server.
For more information, see
vnc_Viewer_getPeerAddress()
.Throws: vncsdk.VncException
on errorReturn type: string
-
Viewer.
getPictureQuality
()¶ Returns the viewer’s current picture quality.
For more information, see
vnc_Viewer_getPictureQuality()
.Return type: vncsdk.Viewer.PictureQuality
-
Viewer.
getServerPointerFbData
()¶ Gets the current mouse cursor image from the Server for rendering locally.
For more information, see
vnc_Viewer_getServerPointerFbData()
.Throws: vncsdk.VncException
on errorReturn type: vncsdk.DataBuffer
-
Viewer.
getServerPointerFbHeight
()¶ Gets the height of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbHeight()
.Return type: number
-
Viewer.
getServerPointerFbPixelFormat
()¶ Gets the pixel format of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbPixelFormat()
.Throws: vncsdk.VncException
on errorReturn type: vncsdk.PixelFormat
-
Viewer.
getServerPointerFbWidth
()¶ Gets the width of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbWidth()
.Return type: number
-
Viewer.
getServerPointerXOffset
()¶ Gets the X offset of the “hotspot” of the pointer.
For more information, see
vnc_Viewer_getServerPointerXOffset()
.Return type: number
-
Viewer.
getServerPointerYOffset
()¶ Gets the Y offset of the “hotspot” of the pointer.
For more information, see
vnc_Viewer_getServerPointerYOffset()
.Return type: number
-
Viewer.
getViewerFbHeight
()¶ Gets the height of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbHeight()
.Return type: number
-
Viewer.
getViewerFbPixelFormat
()¶ Gets the pixel format of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbPixelFormat()
.Throws: vncsdk.VncException
on errorReturn type: vncsdk.PixelFormat
-
Viewer.
getViewerFbStride
()¶ Returns the stride of the viewer framebuffer data in pixels, that is, the number of pixels from the start of each row until the start of the next.
For more information, see
vnc_Viewer_getViewerFbStride()
.Return type: number
-
Viewer.
getViewerFbWidth
()¶ Gets the width of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbWidth()
.Return type: number
-
Viewer.
releaseAllKeys
()¶ Send key up events for all currently pressed keys.
For more information, see
vnc_Viewer_releaseAllKeys()
.Throws: vncsdk.VncException
on error
-
Viewer.
sendAuthenticationResponse
(ok, user, passwd)¶ Provides the SDK with the result of a username/password request.
For more information, see
vnc_Viewer_sendAuthenticationResponse()
.Arguments: - ok (boolean) –
- user (string or null) –
- passwd (string or null) –
Throws: vncsdk.VncException
on error
-
Viewer.
sendClipboardText
(text)¶ Copies the given text to the server’s clipboard.
For more information, see
vnc_Viewer_sendClipboardText()
.Arguments: - text (string) –
Throws: vncsdk.VncException
on error
-
Viewer.
sendKeyDown
(keysym, keyCode)¶ Sends a key down (press) event to the server.
For more information, see
vnc_Viewer_sendKeyDown()
.Arguments: - keysym (number) –
- keyCode (number) –
Throws: vncsdk.VncException
on error
-
Viewer.
sendKeyUp
(keyCode)¶ Sends a key up (release) event to the server.
For more information, see
vnc_Viewer_sendKeyUp()
.Arguments: - keyCode (number) –
Throws: vncsdk.VncException
on error
-
Viewer.
sendPeerVerificationResponse
(ok)¶ Provides the SDK with the response to the
Viewer.PeerVerificationCallback.verifyPeer()
request.For more information, see
vnc_Viewer_sendPeerVerificationResponse()
.Arguments: - ok (boolean) –
Throws: vncsdk.VncException
on error
-
Viewer.
sendPointerEvent
(x, y, buttonState, rel)¶ Sends a pointer event to the server.
For more information, see
vnc_Viewer_sendPointerEvent()
.Arguments: - x (number) –
- y (number) –
- buttonState (Set<
vncsdk.Viewer.MouseButton
>) – - rel (boolean) –
Throws: vncsdk.VncException
on error
-
Viewer.
sendScrollEvent
(delta, axis)¶ Sends a scroll wheel event to the server.
For more information, see
vnc_Viewer_sendScrollEvent()
.Arguments: - delta (number) –
- axis (
vncsdk.Viewer.MouseWheel
) –
Throws: vncsdk.VncException
on error
-
Viewer.
setAuthenticationCallback
(callback)¶ Sets the callback to be called when a username and/or password is required.
For more information, see
vnc_Viewer_setAuthenticationCallback()
.Arguments: - callback (
vncsdk.Viewer.AuthenticationCallback
or null) –
Throws: vncsdk.VncException
on error- callback (
-
Viewer.
setConnectionCallback
(callback)¶ Sets the callbacks for the Viewer to call when various events occur during its lifetime.
For more information, see
vnc_Viewer_setConnectionCallback()
.Arguments: - callback (
vncsdk.Viewer.ConnectionCallback
or null) –
Throws: vncsdk.VncException
on error- callback (
-
Viewer.
setEncryptionLevel
(level)¶ Sets the desired encryption level of the session from the range of options enumerated by :js:class:`vncsdk.Viewer`_EncryptionLevel.
For more information, see
vnc_Viewer_setEncryptionLevel()
.Arguments: - level (
vncsdk.Viewer.EncryptionLevel
) –
Throws: vncsdk.VncException
on error- level (
-
Viewer.
setFramebufferCallback
(callback)¶ Sets the framebuffer callback for this viewer.
For more information, see
vnc_Viewer_setFramebufferCallback()
.Arguments: - callback (
vncsdk.Viewer.FramebufferCallback
or null) –
Throws: vncsdk.VncException
on error- callback (
-
Viewer.
setPeerVerificationCallback
(callback)¶ Sets the callbacks to be called to verify the identity of the peer (server).
For more information, see
vnc_Viewer_setPeerVerificationCallback()
.Arguments: - callback (
vncsdk.Viewer.PeerVerificationCallback
or null) –
Throws: vncsdk.VncException
on error- callback (
-
Viewer.
setPictureQuality
(quality)¶ Sets the desired picture quality of the session from the range of options enumerated by :js:class:`vncsdk.Viewer`_PictureQuality.
For more information, see
vnc_Viewer_setPictureQuality()
.Arguments: - quality (
vncsdk.Viewer.PictureQuality
) –
Throws: vncsdk.VncException
on error- quality (
-
Viewer.
setServerEventCallback
(callback)¶ Sets the server event callback for this viewer.
For more information, see
vnc_Viewer_setServerEventCallback()
.Arguments: - callback (
vncsdk.Viewer.ServerEventCallback
or null) –
Throws: vncsdk.VncException
on error- callback (
-
Viewer.
setServerPointerCallback
(callback)¶ Sets the server pointer callback for this viewer.
For more information, see
vnc_Viewer_setServerPointerCallback()
.Arguments: - callback (
vncsdk.Viewer.ServerPointerCallback
or null) –
Throws: vncsdk.VncException
on error- callback (
-
Viewer.
setServerPointerFbPixelFormat
(pf)¶ Sets the pixel format of the pointer framebuffer.
For more information, see
vnc_Viewer_setServerPointerFbPixelFormat()
.Arguments: - pf (
vncsdk.PixelFormat
) –
- pf (
-
Viewer.
setServerPointerPosPeriod
(periodMs)¶ Sets the rate with which serverPointerPos callback will be called.
For more information, see
vnc_Viewer_setServerPointerPosPeriod()
.Arguments: - periodMs (number) –
-
Viewer.
setViewerFb
(pf, width, height, stride)¶ Sets the viewer framebuffer.
For more information, see
vnc_Viewer_setViewerFb()
.Arguments: - pf (
vncsdk.PixelFormat
) – - width (number) –
- height (number) –
- stride (number) –
Throws: vncsdk.VncException
on error- pf (