Learn how to evaluate and integrate the VNC SDK
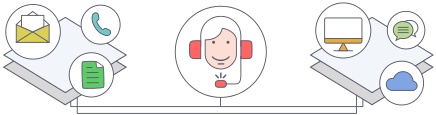
Viewer¶
-
class Viewer
¶ A VNC-compatible Viewer showing the screen of and controlling a remote computer.
For more information, see
vnc_Viewer
in the C API documentation.
Implements¶
Nested classes¶
- Viewer.ConnectionCallback
- Viewer.FramebufferCallback
- Viewer.ServerEventCallback
- Viewer.ServerPointerCallback
- Viewer.AuthenticationCallback
- Viewer.PeerVerificationCallback
- Viewer.ConnectionStatus
- Viewer.DisconnectFlags
- Viewer.MouseButton
- Viewer.MouseWheel
- Viewer.PictureQuality
- Viewer.Encoding
- Viewer.EncryptionLevel
Constructor summary
Modifier and Type | Method and Description |
---|---|
Creates and returns a new viewer. |
Method summary
Modifier and Type | Method and Description |
---|---|
void |
Destroys the viewer. |
void |
Sets the callbacks for the Viewer to call when various events occur during its lifetime. |
ConnectionHandler |
Returns the viewer’s |
Viewer.ConnectionStatus |
Returns the status of the viewer’s connection. |
String |
Returns the address of the viewer’s server. |
void |
Disconnects this viewer from the server. |
String |
Returns a string ID representing the reason for the last viewer disconnection. |
String |
Returns a human-readable message sent by the server for the last disconnection, or null if the last disconnection was not initiated by the server. |
void |
Sets the framebuffer callback for this viewer. |
void |
Sets the viewer framebuffer. |
Int32 |
Gets the width of the viewer framebuffer. |
Int32 |
Gets the height of the viewer framebuffer. |
ImmutablePixelFormat |
Gets the pixel format of the viewer framebuffer. |
ImmutableDataBuffer |
Returns the viewer framebuffer data for the given rectangle. |
Int32 |
Returns the stride of the viewer framebuffer data in pixels, that is, the number of pixels from the start of each row until the start of the next. |
void |
Sets the server event callback for this viewer. |
void |
Copies the given text to the server’s clipboard. |
void |
Sets the server pointer callback for this viewer. |
void |
Sets the pixel format of the pointer framebuffer. |
void |
Sets the rate with which serverPointerPos callback will be called. |
Int32 |
Gets the width of the pointer framebuffer. |
Int32 |
Gets the height of the pointer framebuffer. |
Int32 |
Gets the X offset of the “hotspot” of the pointer. |
Int32 |
Gets the Y offset of the “hotspot” of the pointer. |
ImmutablePixelFormat |
Gets the pixel format of the pointer framebuffer. |
ImmutableDataBuffer |
Gets the current mouse cursor image from the Server for rendering locally. |
void |
Sends a pointer event to the server. |
void |
Sends a scroll wheel event to the server. |
void |
Sends a key down (press) event to the server. |
void |
Sends a key up (release) event to the server. |
void |
Send key up events for all currently pressed keys. |
void |
Sets the callback to be called when a username and/or password is required. |
void |
Allows the Viewer to attempt to authenticate without entering a password, if the VNC Server supports this feature and all the necessary network infrastructure is in place. |
void |
Provides the SDK with the result of a username/password request. |
void |
Sets the callbacks to be called to verify the identity of the peer (server). |
void |
Provides the SDK with the response to the Viewer.PeerVerificationCallback::verifyPeer request. |
void |
Enables receipt of audio from the Server by the Viewer. |
AnnotationManager |
Obtains the Viewer’s |
Viewer.PictureQuality |
Returns the viewer’s current picture quality. |
void |
Sets the desired picture quality of the session from the range of options
enumerated by |
Viewer.Encoding |
Gets the currently set encoding, whether automatically determined or set by Viewer.setEncoding(). |
void |
Enables the use of a specific encoding for the session. |
MessagingManager |
Obtains the Viewer’s Messaging Manager for handling messaging |
void |
Sets the desired encryption level of the session from the range of options
enumerated by |
Viewer.EncryptionLevel |
Returns the Viewer’s current encryption level. |
DisplayManager |
Obtains the Viewer’s |
Constructors¶
-
Viewer
()¶ Creates and returns a new viewer.
For more information, see
vnc_Viewer_create()
in the C API documentation.
Methods¶
-
void
Viewer.Dispose
()¶ Destroys the viewer.
For more information, see
vnc_Viewer_destroy()
in the C API documentation.
-
void
Viewer.SetConnectionCallback
(Viewer.ConnectionCallback callback)¶ Sets the callbacks for the Viewer to call when various events occur during its lifetime.
For more information, see
vnc_Viewer_setConnectionCallback()
in the C API documentation.
-
ConnectionHandler
Viewer.GetConnectionHandler
()¶ Returns the viewer’s
ConnectionHandler
for accepting connections.For more information, see
vnc_Viewer_getConnectionHandler()
in the C API documentation.
-
Viewer.ConnectionStatus
Viewer.GetConnectionStatus
()¶ Returns the status of the viewer’s connection.
For more information, see
vnc_Viewer_getConnectionStatus()
in the C API documentation.
-
String
Viewer.GetPeerAddress
()¶ Returns the address of the viewer’s server.
For more information, see
vnc_Viewer_getPeerAddress()
in the C API documentation.
-
void
Viewer.Disconnect
()¶ Disconnects this viewer from the server.
For more information, see
vnc_Viewer_disconnect()
in the C API documentation.
-
String
Viewer.GetDisconnectReason
()¶ Returns a string ID representing the reason for the last viewer disconnection.
For more information, see
vnc_Viewer_getDisconnectReason()
in the C API documentation.
-
String
Viewer.GetDisconnectMessage
()¶ Returns a human-readable message sent by the server for the last disconnection, or null if the last disconnection was not initiated by the server.
For more information, see
vnc_Viewer_getDisconnectMessage()
in the C API documentation.
-
void
Viewer.SetFramebufferCallback
(Viewer.FramebufferCallback callback)¶ Sets the framebuffer callback for this viewer.
For more information, see
vnc_Viewer_setFramebufferCallback()
in the C API documentation.
-
void
Viewer.SetViewerFb
(Byte[] pixels, ImmutablePixelFormat pf, Int32 width, Int32 height, Int32 stride)¶ Sets the viewer framebuffer.
For more information, see
vnc_Viewer_setViewerFb()
in the C API documentation.
-
Int32
Viewer.GetViewerFbWidth
()¶ Gets the width of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbWidth()
in the C API documentation.
-
Int32
Viewer.GetViewerFbHeight
()¶ Gets the height of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbHeight()
in the C API documentation.
-
ImmutablePixelFormat
Viewer.GetViewerFbPixelFormat
()¶ Gets the pixel format of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbPixelFormat()
in the C API documentation.
-
ImmutableDataBuffer
Viewer.GetViewerFbData
(Int32 x, Int32 y, Int32 w, Int32 h)¶ Returns the viewer framebuffer data for the given rectangle.
For more information, see
vnc_Viewer_getViewerFbData()
in the C API documentation.
-
Int32
Viewer.GetViewerFbStride
()¶ Returns the stride of the viewer framebuffer data in pixels, that is, the number of pixels from the start of each row until the start of the next.
For more information, see
vnc_Viewer_getViewerFbStride()
in the C API documentation.
-
void
Viewer.SetServerEventCallback
(Viewer.ServerEventCallback callback)¶ Sets the server event callback for this viewer.
For more information, see
vnc_Viewer_setServerEventCallback()
in the C API documentation.
-
void
Viewer.SendClipboardText
(String text)¶ Copies the given text to the server’s clipboard.
For more information, see
vnc_Viewer_sendClipboardText()
in the C API documentation.
-
void
Viewer.SetServerPointerCallback
(Viewer.ServerPointerCallback callback)¶ Sets the server pointer callback for this viewer.
For more information, see
vnc_Viewer_setServerPointerCallback()
in the C API documentation.
-
void
Viewer.SetServerPointerFbPixelFormat
(ImmutablePixelFormat pf)¶ Sets the pixel format of the pointer framebuffer.
For more information, see
vnc_Viewer_setServerPointerFbPixelFormat()
in the C API documentation.
-
void
Viewer.SetServerPointerPosPeriod
(Int32 periodMs)¶ Sets the rate with which serverPointerPos callback will be called.
For more information, see
vnc_Viewer_setServerPointerPosPeriod()
in the C API documentation.
-
Int32
Viewer.GetServerPointerFbWidth
()¶ Gets the width of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbWidth()
in the C API documentation.
-
Int32
Viewer.GetServerPointerFbHeight
()¶ Gets the height of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbHeight()
in the C API documentation.
-
Int32
Viewer.GetServerPointerXOffset
()¶ Gets the X offset of the “hotspot” of the pointer.
For more information, see
vnc_Viewer_getServerPointerXOffset()
in the C API documentation.
-
Int32
Viewer.GetServerPointerYOffset
()¶ Gets the Y offset of the “hotspot” of the pointer.
For more information, see
vnc_Viewer_getServerPointerYOffset()
in the C API documentation.
-
ImmutablePixelFormat
Viewer.GetServerPointerFbPixelFormat
()¶ Gets the pixel format of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbPixelFormat()
in the C API documentation.
-
ImmutableDataBuffer
Viewer.GetServerPointerFbData
()¶ Gets the current mouse cursor image from the Server for rendering locally.
For more information, see
vnc_Viewer_getServerPointerFbData()
in the C API documentation.
-
void
Viewer.SendPointerEvent
(Int32 x, Int32 y, Viewer.MouseButton buttonState, Boolean rel)¶ Sends a pointer event to the server.
For more information, see
vnc_Viewer_sendPointerEvent()
in the C API documentation.
-
void
Viewer.SendScrollEvent
(Int32 delta, Viewer.MouseWheel axis)¶ Sends a scroll wheel event to the server.
For more information, see
vnc_Viewer_sendScrollEvent()
in the C API documentation.
-
void
Viewer.SendKeyDown
(Int32 keysym, Int32 keyCode)¶ Sends a key down (press) event to the server.
For more information, see
vnc_Viewer_sendKeyDown()
in the C API documentation.
-
void
Viewer.SendKeyUp
(Int32 keyCode)¶ Sends a key up (release) event to the server.
For more information, see
vnc_Viewer_sendKeyUp()
in the C API documentation.
-
void
Viewer.ReleaseAllKeys
()¶ Send key up events for all currently pressed keys.
For more information, see
vnc_Viewer_releaseAllKeys()
in the C API documentation.
-
void
Viewer.SetAuthenticationCallback
(Viewer.AuthenticationCallback callback)¶ Sets the callback to be called when a username and/or password is required.
For more information, see
vnc_Viewer_setAuthenticationCallback()
in the C API documentation.
-
void
Viewer.EnableSingleSignOn
(Boolean enable)¶ Allows the Viewer to attempt to authenticate without entering a password, if the VNC Server supports this feature and all the necessary network infrastructure is in place.
For more information, see
vnc_Viewer_enableSingleSignOn()
in the C API documentation.
-
void
Viewer.SendAuthenticationResponse
(Boolean ok, String user, String passwd)¶ Provides the SDK with the result of a username/password request.
For more information, see
vnc_Viewer_sendAuthenticationResponse()
in the C API documentation.
-
void
Viewer.SetPeerVerificationCallback
(Viewer.PeerVerificationCallback callback)¶ Sets the callbacks to be called to verify the identity of the peer (server).
For more information, see
vnc_Viewer_setPeerVerificationCallback()
in the C API documentation.
-
void
Viewer.SendPeerVerificationResponse
(Boolean ok)¶ Provides the SDK with the response to the Viewer.PeerVerificationCallback::verifyPeer request.
For more information, see
vnc_Viewer_sendPeerVerificationResponse()
in the C API documentation.
-
void
Viewer.EnableAudio
(Boolean enable)¶ Enables receipt of audio from the Server by the Viewer.
For more information, see
vnc_Viewer_enableAudio()
in the C API documentation.
-
AnnotationManager
Viewer.GetAnnotationManager
()¶ Obtains the Viewer’s
AnnotationManager
for handling annotation operations.For more information, see
vnc_Viewer_getAnnotationManager()
in the C API documentation.
-
Viewer.PictureQuality
Viewer.GetPictureQuality
()¶ Returns the viewer’s current picture quality.
For more information, see
vnc_Viewer_getPictureQuality()
in the C API documentation.
-
void
Viewer.SetPictureQuality
(Viewer.PictureQuality quality)¶ Sets the desired picture quality of the session from the range of options enumerated by
Viewer.PictureQuality
.For more information, see
vnc_Viewer_setPictureQuality()
in the C API documentation.
-
Viewer.Encoding
Viewer.GetEncoding
()¶ Gets the currently set encoding, whether automatically determined or set by Viewer.setEncoding().
For more information, see
vnc_Viewer_getEncoding()
in the C API documentation.
-
void
Viewer.SetEncoding
(Viewer.Encoding encoding)¶ Enables the use of a specific encoding for the session.
For more information, see
vnc_Viewer_setEncoding()
in the C API documentation.
-
MessagingManager
Viewer.GetMessagingManager
()¶ Obtains the Viewer’s Messaging Manager for handling messaging
For more information, see
vnc_Viewer_getMessagingManager()
in the C API documentation.
-
void
Viewer.SetEncryptionLevel
(Viewer.EncryptionLevel level)¶ Sets the desired encryption level of the session from the range of options enumerated by
Viewer.EncryptionLevel
.For more information, see
vnc_Viewer_setEncryptionLevel()
in the C API documentation.
-
Viewer.EncryptionLevel
Viewer.GetEncryptionLevel
()¶ Returns the Viewer’s current encryption level.
For more information, see
vnc_Viewer_getEncryptionLevel()
in the C API documentation.
-
DisplayManager
Viewer.GetDisplayManager
()¶ Obtains the Viewer’s
DisplayManager
, for managing the list of displays made available by the Server to the Viewer.For more information, see
vnc_Viewer_getDisplayManager()
in the C API documentation.