Learn how to evaluate and integrate the VNC SDK
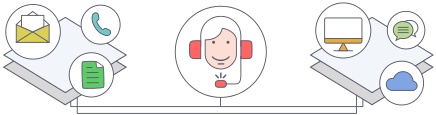
.NET API¶
The VNC SDK provides .NET bindings that enable you to create a VNC Viewer or a VNC Server app in a .NET language.
When using the .NET bindings, the .NET method calls used to invoke the SDK are implemented using calls into the lower-level C API.
Therefore, to distribute your .NET app you will need to include not only the bindings themselves (RealVNC.VncSdk.dll
) but
also the SDK’s native bindings (vncsdk.dll
). A Server app also requires vncagent.exe
and vncannotator.exe
.
The .NET bindings support the .NET 4.0 framework upwards.
Importing the bindings
Add RealVNC.VncSdk.dll
as a reference to your project and then import it:
using RealVNC.VncSdk;
In order for the .NET bindings to find the SDK the supporting DLLs need to be available for the bindings to load, either by
locating them in a directory with the executable or by supplying the location when calling DynamicLoader.LoadLibrary
.
Deployed production apps should ensure the directory supplied is a trusted directory.
Using the documentation
The primary source for the SDK documentation is provided by the C API reference. Most methods available via the .NET bindings are documented with a brief summary only, since the full behavior and description of each method and argument is contained in the C API reference. This documentation for the .NET bindings describes only the behavior that is unique to the .NET bindings.
Methods and their types are mapped into .NET as follows:
- Each structure in the C API is mapped, along with the relevant functions, to a corresponding .NET class. For example, the
vnc_Server
C API type corresponds to the .NET binding classRealVNC.VncSdk.Server
and the functionvnc_Server_setFriendlyName
is mapped toServer.SetFriendlyName()
. The constructors of .NET classes correspond tovnc...create
methods and theDispose
methods tovnc...destroy
methods in the C API. - Arguments are mapped to their native .NET types. Pass in a .NET string where the C API uses a
const char*
. - The .NET bindings throw exceptions on error where the C API would return
vnc_failure
or NULL and then setvnc_getLastError
. There is no equivalent ‘get last error’ function because aRealVNC.VncSdk.VncException
is thrown for all error cases instead. TheErrorCode
member contains the strings described in the C API reference.
Memory management
It is recommended that you dispose of objects appropriately to ensure good clean up.
Threading
The VNC SDK needs to run in a single thread for the duration of the process, including calls to Library.Init()
and
Library.Shutdown()
. Note that the WPF sample has an implementation of a single-thread library in the VncLibraryThread
class.
All SDK methods need to be called from the SDK thread, with two exceptions: EventLoop.Stop()
and EventLoop.RunOnLoop()
.
The EventLoop
class is a helper class to make it easier to interact with the SDK thread. If you run EventLoop.Run()
on
the background SDK thread then any other thread can call EventLoop.RunOnLoop()
to pass Actions to run on the background thread.
EventLoop.Stop()
will cause the event loop to stop.
Callbacks
The simplest way to define a callback is to pass delegates into the given callback class, for example:
Viewer.SetServerEventCallback(new Viewer.ServerEventCallback((v,name) => Console.Write(name)));
This structure has been used to mirror the C API.