Learn how to evaluate and integrate the VNC SDK
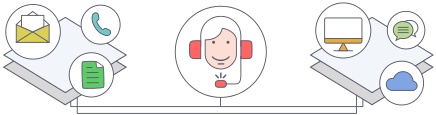
Annotation.h¶
Enables a Viewer or Server to annotate (that is, draw lines on top of) a Server device screen, without affecting the underlying desktop. (more...)
Data structures
Modifier and Type | Name and Description |
---|---|
struct | vnc_AnnotationManager_Callback Callback receiving annotation-related notifications. |
Functions
Modifier and Type | Name and Description |
---|---|
function vnc_status_t | vnc_AnnotationManager_setCallback(vnc_AnnotationManager *annotationManager, const vnc_AnnotationManager_Callback *callback, void *userData) Sets annotation-related callbacks. |
function vnc_status_t | vnc_AnnotationManager_clear(vnc_AnnotationManager *annotationManager, vnc_bool_t fade, vnc_Connection *connection) Clears particular annotations. |
function void | vnc_AnnotationManager_clearAll(vnc_AnnotationManager *annotationManager, vnc_bool_t fade) Clears all annotations. |
function vnc_bool_t | vnc_AnnotationManager_isAvailable(vnc_AnnotationManager *annotationManager) Queries whether it is possible to annotate. |
function vnc_uint32_t | vnc_AnnotationManager_getPenColor(vnc_AnnotationManager *annotationManager) Gets the current pen color. |
function void | vnc_AnnotationManager_setPenColor(vnc_AnnotationManager *annotationManager, vnc_uint32_t color) Sets the pen color, determining the color of the annotation line. |
function vnc_uint31_t | vnc_AnnotationManager_getPenSize(vnc_AnnotationManager *annotationManager) Gets the current pen size. |
function void | vnc_AnnotationManager_setPenSize(vnc_AnnotationManager *annotationManager, vnc_uint31_t size) Sets the pen size, determining the width of the annotation line. |
function int | vnc_AnnotationManager_getPersistDuration(vnc_AnnotationManager *annotationManager) Gets how long annotations persist as a solid color for. |
function void | vnc_AnnotationManager_setPersistDuration(vnc_AnnotationManager *annotationManager, int durationMs) Sets how long annotations persist as a solid color for. |
function int | vnc_AnnotationManager_getFadeDuration(vnc_AnnotationManager *annotationManager) Gets how long annotations take to fade. |
function void | vnc_AnnotationManager_setFadeDuration(vnc_AnnotationManager *annotationManager, int durationMs) Sets how long annotations take to fade. |
function vnc_status_t | vnc_AnnotationManager_movePenTo(vnc_AnnotationManager *annotationManager, int x, int y, vnc_bool_t penDown) Draws a line on the Server screen from the current position to a new position. |
Detailed description
Enables a Viewer or Server to annotate (that is, draw lines on top of) a Server device screen, without affecting the underlying desktop.
To enable a Viewer to annotate, call vnc_Viewer_getAnnotationManager to obtain a vnc_AnnotationManager object. To enable a Server to manage annotations for Viewers, or to annotate itself if desired, call vnc_Server_getAnnotationManager.
A Server must grant a connecting Viewer vnc_Server_PermAnnotation in the set of permissions returned by the vnc_Server_SecurityCallback::authenticateUser callback. A Viewer can call vnc_AnnotationManager_setCallback and monitor vnc_AnnotationManager_Callback::availabilityChanged to be notified if annotation availability changes; this might be due to a transient error, but it might be because the Server has revoked annotation permissions mid-session using vnc_Server_setPermissions.
A vnc_AnnotationManager controls a pen with a unique color determined by the SDK and a sensible default size, duration and fade time. You can query and change these defaults at any time.
Typically, a Viewer or Server annotates in response to an app user moving the mouse cursor or touching the screen. Monitor platform mouse or touch events as appropriate and call vnc_AnnotationManager_movePenTo with penDown
set to vnc_true to draw a line from the current position to a new position. To stop drawing, call the same method with penDown
set to vnc_false. Note a Viewer might want to suspend calls to vnc_Viewer_sendPointerEvent for the duration in order to stop remote control while drawing operations are in progress.
A Viewer can clear its own annotations, or a Server can clear Viewer annotations, or just its own. Note a Viewer’s annotations are automatically cleared when it disconnects, and all annotations are cleared if the Server screen resolution changes, for example when an Android device rotates.
See the Overview page of the Developer Docs for more information Annotating to a server device screen.
- Since
- 1.4
Functions
-
vnc_status_t
vnc_AnnotationManager_setCallback
(vnc_AnnotationManager *annotationManager, const vnc_AnnotationManager_Callback *callback, void *userData)¶ Sets annotation-related callbacks.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
callback
-The new annotation callback.
- Return Value
InvalidArgument
-callback
is invalid.
-
vnc_status_t
vnc_AnnotationManager_clear
(vnc_AnnotationManager *annotationManager, vnc_bool_t fade, vnc_Connection *connection)¶ Clears particular annotations.
For a Viewer, this is all its annotations. For a Server, you can choose to clear its annotations, or those of a particular Viewer.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Since
- 1.4
- Parameters
- Return Value
InvalidArgument
-annotationManager
is invalid orconnection
is invalid.
-
void
vnc_AnnotationManager_clearAll
(vnc_AnnotationManager *annotationManager, vnc_bool_t fade)¶ Clears all annotations.
For a Server, clears all annotations, including its own. For a Viewer, this is the same as vnc_AnnotationManager_clear.
-
vnc_bool_t
vnc_AnnotationManager_isAvailable
(vnc_AnnotationManager *annotationManager)¶ Queries whether it is possible to annotate.
Note that a Server cannot annotate until app initialization is complete. A Viewer cannot annotate if it is not connected to a Server, if it has not been granted annotation permissions, or if the Server does not support annotations (perhaps because that Server was not created using the VNC SDK).
- See
- vnc_AnnotationManager_Callback::availabilityChanged
- Return
- vnc_true if it is possible to annotate, otherwise vnc_false.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
-
vnc_uint32_t
vnc_AnnotationManager_getPenColor
(vnc_AnnotationManager *annotationManager)¶ Gets the current pen color.
- Return
- A 32-bit integer in the form AARRGGBB.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
-
void
vnc_AnnotationManager_setPenColor
(vnc_AnnotationManager *annotationManager, vnc_uint32_t color)¶ Sets the pen color, determining the color of the annotation line.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
color
-A 32-bit integer in the form AARRGGBB.
-
vnc_uint31_t
vnc_AnnotationManager_getPenSize
(vnc_AnnotationManager *annotationManager)¶ Gets the current pen size.
Defaults to 10 device independent pixels (DIP).
- Return
- A number of DIP.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
-
void
vnc_AnnotationManager_setPenSize
(vnc_AnnotationManager *annotationManager, vnc_uint31_t size)¶ Sets the pen size, determining the width of the annotation line.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
size
-A number of DIP.
-
int
vnc_AnnotationManager_getPersistDuration
(vnc_AnnotationManager *annotationManager)¶ Gets how long annotations persist as a solid color for.
Defaults to 10000 milliseconds.
- Return
- A number of milliseconds. A value of 0 means annotations start fading immediately. A value of -1 or lower means annotations persist indefinitely unless explicitly or automatically cleared (see the detailed description summary for when this might happen).
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
-
void
vnc_AnnotationManager_setPersistDuration
(vnc_AnnotationManager *annotationManager, int durationMs)¶ Sets how long annotations persist as a solid color for.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
durationMs
-A number of milliseconds. Specify 0 to start fading immedaitely. Specify -1 or lower to persist annotations indefinitely unless explicitly or automatically cleared (see the detailed description for when this might happen).
-
int
vnc_AnnotationManager_getFadeDuration
(vnc_AnnotationManager *annotationManager)¶ Gets how long annotations take to fade.
Defaults to 2000 milliseconds.
- Return
- A number of milliseconds. A value of 0 means annotations do not fade but rather disappear immediately after vnc_AnnotationManager_getPersistDuration.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
-
void
vnc_AnnotationManager_setFadeDuration
(vnc_AnnotationManager *annotationManager, int durationMs)¶ Sets how long annotations take to fade.
- Since
- 1.4
- Parameters
annotationManager
-Belonging to either a Viewer or a Server.
durationMs
-A number of milliseconds. Specify 0 to clear annotations immediately after vnc_AnnotationManager_getPersistDuration.
-
vnc_status_t
vnc_AnnotationManager_movePenTo
(vnc_AnnotationManager *annotationManager, int x, int y, vnc_bool_t penDown)¶ Draws a line on the Server screen from the current position to a new position.
When you obtain a vnc_AnnotationManager, its co-ordinate system is set to 0,0. This means that before you annotate for the first time, call this method with
penDown
set to vnc_false to move the pen from 0,0 to the current position without drawing a line. You can then call this method again withpenDown
set to vnc_true to actually draw from the current position to a new position. Call this method withpenDown
set to vnc_false to stop drawing.A vnc_AnnotationManager subsequently remembers its current position, so you only need manoevre from 0,0 once. It is only reset to 0,0 if you obtain a new vnc_AnnotationManager, or if the Server screen resolution changes.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- See
- vnc_AnnotationManager_isAvailable
- Since
- 1.4
- Parameters
- Return Value
InvalidArgument
-annotationManager
is invalid.NotSupported
-An attempt was made to annotate with the pen down while annotations were not available.
-
struct
vnc_AnnotationManager_Callback
¶ - #include <Annotation.h>
Callback receiving annotation-related notifications.
Public Members
-
void(* vnc_AnnotationManager_Callback::availabilityChanged) (void *userData, vnc_AnnotationManager *annotationManager, vnc_bool_t isAvailable)
Notification that annotation availability has changed.
This optional callback is notified if a call to vnc_AnnotationManager_isAvailable would return a different value.
-