Learn how to evaluate and integrate the VNC SDK
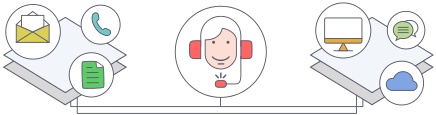
Cloud.h¶
Listens for or establishes connections using VNC Cloud.
Data structures
Modifier and Type | Name and Description |
---|---|
struct |
Callback for a vnc_CloudListener. |
struct | vnc_CloudAddressMonitor_Callback Callback for a vnc_CloudAddressMonitor. |
Enums
Modifier and Type | Name and Description |
---|---|
enum |
Enumeration of listening statuses. |
enum | vnc_CloudAddressMonitor_Availability Enumeration of availabilities for a Cloud address. |
Typedefs
Modifier and Type | Name and Description |
---|---|
typedef struct vnc_CloudListener |
Listener used to join VNC Cloud and listen for a connection. |
typedef struct vnc_CloudConnector |
Connector used to join VNC Cloud and establish an outgoing connection. |
typedef struct vnc_CloudAddressMonitor |
Monitor used to query the availability of VNC Cloud addresses. |
Functions
Modifier and Type | Name and Description |
---|---|
function vnc_CloudListener * | vnc_CloudListener_create(const char *localCloudAddress, const char *localCloudPassword, vnc_ConnectionHandler *connectionHandler, const vnc_CloudListener_Callback *callback, void *userData) Begins listening for incoming connections on the given Cloud address. |
function void | vnc_CloudListener_destroy(vnc_CloudListener *listener) Destroys the Cloud listener. |
function vnc_CloudConnector * | vnc_CloudConnector_create(const char *localCloudAddress, const char *localCloudPassword) Creates a connector, which is used used to create connections to Cloud addresses. |
function void | vnc_CloudConnector_destroy(vnc_CloudConnector *connector) Destroys the Cloud connector. |
function vnc_status_t | vnc_CloudConnector_connect(vnc_CloudConnector *connector, const char *peerCloudAddress, vnc_ConnectionHandler *connectionHandler) Begins an outgoing connection to the given Cloud address. |
function void | vnc_CloudConnector_setWaitForPeer(vnc_CloudConnector *connector, vnc_bool_t waitForPeer) Sets whether new connections created by the connector wait for the peer to start listening. |
function vnc_status_t | vnc_CloudConnector_setRelayBandwidthLimit(vnc_CloudConnector *connector, int relayBandwidthLimit) Set the bandwidth limit applied to relayed Cloud connections. |
function vnc_CloudAddressMonitor * | vnc_CloudAddressMonitor_create(vnc_CloudConnector *connector, const char **cloudAddresses, int nAddresses, const vnc_CloudAddressMonitor_Callback *callback, void *userData) Creates a monitor, which queries whether a list of cloud addresses is available. |
function void | vnc_CloudAddressMonitor_destroy(vnc_CloudAddressMonitor *monitor) Destroys the Cloud monitor. |
function void | vnc_CloudAddressMonitor_pause(vnc_CloudAddressMonitor *monitor) Pauses the Cloud monitor. |
function void | vnc_CloudAddressMonitor_resume(vnc_CloudAddressMonitor *monitor) Resumes the Cloud monitor. |
function void | vnc_CloudAddressMonitor_setPauseOnConnect(vnc_CloudAddressMonitor *monitor, vnc_bool_t pauseOnConnect) Sets whether or not the Cloud monitor pauses automatically when a connection is established. |
function vnc_status_t | vnc_setCloudProxySettings(vnc_bool_t systemProxy, const char *proxyUrl) Specifies proxy server settings for Cloud connections; note these settings are adopted for all subsequent outgoing Cloud connections. |
Detailed description
Listens for or establishes connections using VNC Cloud.
Typedefs
-
typedef struct vnc_CloudListener
vnc_CloudListener
¶ Listener used to join VNC Cloud and listen for a connection.
-
typedef struct vnc_CloudConnector
vnc_CloudConnector
¶ Connector used to join VNC Cloud and establish an outgoing connection.
-
typedef struct vnc_CloudAddressMonitor
vnc_CloudAddressMonitor
¶ Monitor used to query the availability of VNC Cloud addresses.
Enums
-
enum
vnc_CloudListener_Status
¶ Enumeration of listening statuses.
Values:
-
vnc_CloudListener_StatusSearching
¶ The listener is in the process of establishing an association with VNC Cloud.
Incoming connections are not yet possible.
-
vnc_CloudListener_StatusOnline
¶ The listener is available for incoming connections.
-
-
enum
vnc_CloudAddressMonitor_Availability
¶ Enumeration of availabilities for a Cloud address.
Values:
-
vnc_CloudAddressMonitor_Available
¶ A peer is currently listening on the Cloud address.
No peer is currently listening on the Cloud address.
-
vnc_CloudAddressMonitor_UnknownAvailability
¶ The Cloud address monitor is waiting for the Cloud services to return the availability for the peer address, or an error occurred.
-
Functions
-
vnc_CloudListener *
vnc_CloudListener_create
(const char *localCloudAddress, const char *localCloudPassword, vnc_ConnectionHandler *connectionHandler, const vnc_CloudListener_Callback *callback, void *userData)¶ Begins listening for incoming connections on the given Cloud address.
To stop listening, destroy the Cloud listener.
The Cloud listener’s status is initially vnc_CloudListener_StatusSearching while it is locating a nearby Cloud server to use, then changes to vnc_CloudListener_StatusOnline once it is ready to receive connections; when this happens the vnc_CloudListener_Callback::listeningStatusChanged function is called. It is also possible for the listener to briefly switch back to vnc_CloudListener_StatusSearching during normal operation.
If an error occurs, then the listener reaches a terminal error state; when this happens, the vnc_CloudListener_Callback::listeningFailed function is called and the vnc_CloudListener_Callback::listeningStatusChanged function is not notified. The only thing that can be done after an error is to destroy the listener and create a new one after a delay.
- Return
- Returns a new vnc_CloudListener on success, or NULL on error. If an error is returned, vnc_getLastError() can be used to get the error code.
- Parameters
localCloudAddress
-The cloud address on which to listen for incoming connections (typically a string with four components of the form
XXX.XXX.XXX.XXX
).localCloudPassword
-The password associated with
localCloudAddress
(typically a short alphanumeric string).connectionHandler
-The object which shall handle incoming connections (if not rejected by the filter function). It is either a server or a viewer. It must be destroyed after the Cloud listener.
callback
-The callback is required, in order to receive notification of errors.
- Return Value
InvalidArgument
-The given Cloud address and password are malformed
AddressError
-The given Cloud address is not valid or is already in use
-
void
vnc_CloudListener_destroy
(vnc_CloudListener *listener)¶ Destroys the Cloud listener.
Any ongoing connections are not affected, but new connections will not be accepted.
- Parameters
listener
-The listener.
-
vnc_CloudConnector *
vnc_CloudConnector_create
(const char *localCloudAddress, const char *localCloudPassword)¶ Creates a connector, which is used used to create connections to Cloud addresses.
- Return
- Returns a new vnc_CloudConnector on success, or NULL on error. If an error is returned, vnc_getLastError() can be used to get the error code.
- Parameters
localCloudAddress
-The cloud address representing our end for outgoing connections (typically a string with four components of the form
XXX.XXX.XXX.XXX
).localCloudPassword
-The password associated with
localCloudAddress
(typically a short alphanumeric string).
- Return Value
InvalidArgument
-The given Cloud address or password is malformed
AddressError
-The given Cloud address is not valid or is already in use
-
void
vnc_CloudConnector_destroy
(vnc_CloudConnector *connector)¶ Destroys the Cloud connector.
- Parameters
connector
-The connector.
-
vnc_status_t
vnc_CloudConnector_connect
(vnc_CloudConnector *connector, const char *peerCloudAddress, vnc_ConnectionHandler *connectionHandler)¶ Begins an outgoing connection to the given Cloud address.
The connection will be handled by the
connectionHandler
.The Cloud connector can be used to create connections to any number of different peer addresses, without having to wait for earlier connections to finish (note however that a viewer cannot be used as a connection handler if it is already connected). Two simultaneous connections to the same peer Cloud address are not supported. The connector can be destroyed immediately after creating a connection, without affecting the connection: the connection handler immediately takes ownership of it.
Connection errors are notified using the server or viewer’s callbacks (depending on the type of the connection handler).
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Parameters
connector
-The connector.
peerCloudAddress
-The cloud address to make a connection to.
connectionHandler
-The object which shall handle the outgoing connection. It is either a server or a viewer. Connection errors are notified via the connection handler’s callbacks.
- Return Value
InvalidArgument
-The given Cloud address or password is malformed
UnexpectedCall
-The connection handler is already connected
-
void
vnc_CloudConnector_setWaitForPeer
(vnc_CloudConnector *connector, vnc_bool_t waitForPeer)¶ Sets whether new connections created by the connector wait for the peer to start listening.
The default behaviour is for connections to fail if the peer is not currently listening.
- Parameters
connector
-The connector.
waitForPeer
-If set to vnc_true, then the connection attempt will wait for the peer to create a Cloud listener. The application must decide how long to wait, and cancel the connection attempt after a suitable timeout by destroying the viewer.
-
vnc_status_t
vnc_CloudConnector_setRelayBandwidthLimit
(vnc_CloudConnector *connector, int relayBandwidthLimit)¶ Set the bandwidth limit applied to relayed Cloud connections.
Connections made via VNC Cloud are either direct or relayed. A direct connection will be made where possible, but if one of the peers is behind a restrictive NAT or firewall, then VNC Cloud will be used to relay the data. In this case, a bandwidth limit may be set to control use of the relay.
After calling vnc_CloudConnector_create(), the connector’s initial bandwidth limit is unlimited. If a new limit is set on the connector, it will be applied to all subsequent connections started using vnc_CloudConnector_connect().
- Return
vnc_success
is returned on success orvnc_failure
in the case of an error, in which case vnc_getLastError() can be used to get the error code.- Parameters
relayBandwidthLimit
-The limit in bytes per second, or -1 for unlimited. The allowed values are in the range 1024 to 999999999.
- Return Value
InvalidArgument
-The bandwidth limit is out of range
-
vnc_CloudAddressMonitor *
vnc_CloudAddressMonitor_create
(vnc_CloudConnector *connector, const char **cloudAddresses, int nAddresses, const vnc_CloudAddressMonitor_Callback *callback, void *userData)¶ Creates a monitor, which queries whether a list of cloud addresses is available.
(An address is “available” if there is a Cloud listener currently accepting connections on that address.) The monitor will notify its callback whenever the availability information for an address is updated.
Each Cloud connector can have at most one associated monitor at one time.
- Return
- Returns a new vnc_CloudAddressMonitor on success, or NULL on error. If an error is returned, vnc_getLastError() can be used to get the error code.
- Parameters
connector
-The connector used by the monitor for queries to VNC Cloud.
cloudAddresses
-An array of cloud addresses to be monitored.
nAddresses
-The length of the
cloudAddresses
array.callback
-The required callback for receiving availability information.
- Return Value
UnexpectedCall
-The given Cloud connector already has a monitor
InvalidArgument
-One of the given Cloud addresses is malformed
-
void
vnc_CloudAddressMonitor_destroy
(vnc_CloudAddressMonitor *monitor)¶ Destroys the Cloud monitor.
- Parameters
monitor
-The monitor.
-
void
vnc_CloudAddressMonitor_pause
(vnc_CloudAddressMonitor *monitor)¶ Pauses the Cloud monitor.
While paused, updates will not be fetched for the peer addresses, however, the callback may still be notified of changes in availability for a short while after pausing. If a list of cloud addresses is being shown with their availabilities, it would be appropriate to pause the monitor while the window is not focused, for example.
- Parameters
monitor
-The monitor.
-
void
vnc_CloudAddressMonitor_resume
(vnc_CloudAddressMonitor *monitor)¶ Resumes the Cloud monitor.
- Parameters
monitor
-The monitor.
-
void
vnc_CloudAddressMonitor_setPauseOnConnect
(vnc_CloudAddressMonitor *monitor, vnc_bool_t pauseOnConnect)¶ Sets whether or not the Cloud monitor pauses automatically when a connection is established.
Normally, a Cloud monitor does not need to run during a connection, so the default behaviour is to reduce bandwidth by pausing the monitor when a connection is established. If
pauseOnConnect
is set to vnc_false, the user must be careful to pause the monitor when possible, rather than continuously polling the network.- Parameters
monitor
-The monitor.
pauseOnConnect
-Whether to pause when a new incoming connection is established.
-
vnc_status_t
vnc_setCloudProxySettings
(vnc_bool_t systemProxy, const char *proxyUrl)¶ Specifies proxy server settings for Cloud connections; note these settings are adopted for all subsequent outgoing Cloud connections.
By default, the SDK employs the system configured proxy settings when making Cloud connections. You can specify a particular proxy server, or supply full user credentials. Note that any connection error arising from the proxy settings is reported when an outgoing connection is attempted; see vnc_CloudConnector_connect.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Since
- 1.4
- Parameters
systemProxy
-Set this to vnc_true to employ the currently configured system proxy settings. When set to vnc_false,
proxyUrl
is used to specify the proxy settings.proxyUrl
-A string specifying a proxy server, plus user credentials if required. The format of the string is
type://username:password@address::port
, wheretype
is eithersocks
orhttp
, for examplesocks://fred:X$fr£4Pw@mySocks5Proxy.here.com::8888
. Note that ifsystemProxy
is vnc_false andproxyUrl
is null or empty then this is treated as a request to stop using a proxy server.
- Return Value
InvalidArgument
-proxyUrl
does not conform to the required format (see above).
-
struct
vnc_CloudListener_Callback
¶ - #include <Cloud.h>
Callback for a vnc_CloudListener.
Public Members
-
void(* vnc_CloudListener_Callback::listeningFailed) (void *userData, vnc_CloudListener *listener, const char *cloudError, int retryTimeSecs)
Notification that the Cloud listener has stopped listening and is unable to accept any more connections.
This callback is required.
The Cloud listener internally handles errors due to transient networking conditions, but if a connection to VNC Cloud cannot be promptly re-established, the Cloud listener stops, leaving the application to choose whether to retry or to present an error message to the user. In a long-running application where the user may not be present, it is normal to retry wherever possible; in a short-lived “helpdesk” application it may be desirable not to retry at all when there are errors but simply present a message to the user.
Some errors are unrecoverable, such as invalid Cloud credentials, and in this case the user must be notified rather than creating a new Cloud listener using the same credentials. In this case,
retryTimeSecs
is -1.For recoverable errors (such as a lack of network connectivity), is is possible to retry listening. This is done by destroying the Cloud listener, then creating a new one after a delay. The delay should not be too short, or excessive bandwidth and needless load on the VNC Cloud will be generated. The
retryTimeSecs
parameter indicates a safe delay to use, starting at five seconds and gradually increasing to a maximum with each failure. Do not retry more rapidly than indicated byretryTimeSecs
, or service may be impaired for other users of VNC Cloud at times of peak load.- Parameters
cloudError
-A specific error string describing the cause. Possible errors are:
CloudAuthenticationFailed
,CloudConnectivityError
.retryTimeSecs
-The time in seconds to wait before attempting to listen again using this Cloud address, or -1 if the error is fatal and automatic recovery after a delay is not possible.
-
vnc_bool_t(* vnc_CloudListener_Callback::filterConnection) (void *userData, vnc_CloudListener *listener, const char *peerCloudAddress)
Notification to provide address-based filtering of incoming connections.
This callback is optional.
-
void(* vnc_CloudListener_Callback::listeningStatusChanged) (void *userData, vnc_CloudListener *listener, vnc_CloudListener_Status status)
Notification that the listener status has changed.
This callback is optional.
After creating a listener, it is initially unable to receive connections until it has successfully checked-in with VNC Cloud. If network connectivity is temporarily lost, the status can also change from vnc_CloudListener_StatusOnline back to vnc_CloudListener_StatusSearching.
- Parameters
status
-The new status of the Cloud listener.
-
-
struct
vnc_CloudAddressMonitor_Callback
¶ - #include <Cloud.h>
Callback for a vnc_CloudAddressMonitor.
Public Members
-
void(* vnc_CloudAddressMonitor_Callback::availabilityChanged) (void *userData, vnc_CloudAddressMonitor *monitor, const char *cloudAddress, vnc_CloudAddressMonitor_Availability availability)
Notification that the availability information for a Cloud address has changed.
This callback is required.
- Parameters
cloudAddress
-The address whose availability has changed.
availability
-The new value for the availability.
-
void(* vnc_CloudAddressMonitor_Callback::monitorPaused) (void *userData, vnc_CloudAddressMonitor *monitor)
Notification that monitoring has been paused by an outgoing connection starting.
This callback is optional.
The monitor should not normally need to be restarted in particular, if availability information is not being displayed to the user the monitor should not be resumed.
-