Learn how to evaluate and integrate the VNC SDK
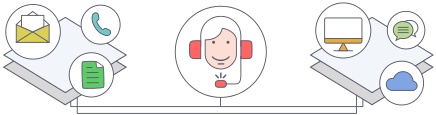
Viewer¶
-
public final class
Viewer
¶ A VNC-compatible Viewer showing the screen of and controlling a remote computer.
Nested Classes¶
- interface
Viewer.AuthenticationCallback
- interface
Viewer.ConnectionCallback
- enum
Viewer.ConnectionStatus
- enum
Viewer.DisconnectFlags
- enum
Viewer.Encoding
- enum
Viewer.EncryptionLevel
- interface
Viewer.FramebufferCallback
- enum
Viewer.MouseButton
- enum
Viewer.MouseWheel
- interface
Viewer.PeerVerificationCallback
- enum
Viewer.PictureQuality
- interface
Viewer.ServerEventCallback
- interface
Viewer.ServerPointerCallback
Method Summary¶
Modifier and Type | Method and Description |
---|---|
void |
Destroys the viewer. |
void |
Disconnects this viewer from the server. |
void |
Enables receipt of audio from the Server by the Viewer. |
void |
Allows the Viewer to attempt to authenticate without entering a password, if the VNC Server supports this feature and all the necessary network infrastructure is in place. |
AnnotationManager |
Obtains the Viewer’s |
ConnectionHandler |
Returns the viewer’s |
ConnectionStatus |
Returns the status of the viewer’s connection. |
String |
Returns a human-readable message sent by the server for the last disconnection, or |
String |
Returns a string ID representing the reason for the last viewer disconnection. |
DisplayManager |
Obtains the Viewer’s |
Encoding |
Gets the currently set encoding, whether automatically determined or set by |
EncryptionLevel |
Returns the Viewer’s current encryption level. |
MessagingManager |
Obtains the Viewer’s Messaging Manager for handling messaging |
String |
Returns the address of the viewer’s server. |
PictureQuality |
Returns the viewer’s current picture quality. |
byte[] |
Gets the current mouse cursor image from the Server for rendering locally. |
int |
Gets the height of the pointer framebuffer. |
ImmutablePixelFormat |
Gets the pixel format of the pointer framebuffer. |
int |
Gets the width of the pointer framebuffer. |
int |
Gets the X offset of the “hotspot” of the pointer. |
int |
Gets the Y offset of the “hotspot” of the pointer. |
byte[] |
Returns the viewer framebuffer data for the given rectangle. |
void |
Draw the framebuffer data into an Android |
int |
Gets the height of the viewer framebuffer. |
ImmutablePixelFormat |
Gets the pixel format of the viewer framebuffer. |
int |
Returns the stride of the viewer framebuffer data in pixels, that is, the number of pixels from the start of each row until the start of the next. |
int |
Gets the width of the viewer framebuffer. |
void |
Send key up events for all currently pressed keys. |
void |
Provides the SDK with the result of a username/password request. |
void |
Copies the given text to the server’s clipboard. |
void |
Sends a key down (press) event to the server. |
void |
Sends a key up (release) event to the server. |
void |
Provides the SDK with the response to the |
void |
Sends a pointer event to the server. |
void |
Sends a scroll wheel event to the server. |
void |
Sets the callback to be called when a username and/or password is required. |
void |
Sets the callbacks for the Viewer to call when various events occur during its lifetime. |
void |
Enables the use of a specific encoding for the session. |
void |
Sets the desired encryption level of the session from the range of options enumerated by |
void |
Sets the framebuffer callback for this viewer. |
void |
Sets the callbacks to be called to verify the identity of the peer (server). |
void |
Sets the desired picture quality of the session from the range of options enumerated by |
void |
Sets the server event callback for this viewer. |
void |
Sets the server pointer callback for this viewer. |
void |
Sets the pixel format of the pointer framebuffer. |
void |
Sets the rate with which serverPointerPos callback will be called. |
void |
Sets the viewer framebuffer. |
Constructors¶
-
public
Viewer
() throws Library.VncException¶ Creates and returns a new viewer.
For more information, see
vnc_Viewer_create()
.
Methods¶
-
public void
destroy
()¶ Destroys the viewer.
For more information, see
vnc_Viewer_destroy()
.
-
public void
disconnect
() throws Library.VncException¶ Disconnects this viewer from the server.
For more information, see
vnc_Viewer_disconnect()
.
-
public void
enableAudio
(boolean enable) throws Library.VncException¶ Enables receipt of audio from the Server by the Viewer.
For more information, see
vnc_Viewer_enableAudio()
.
-
public void
enableSingleSignOn
(boolean enable) throws Library.VncException¶ Allows the Viewer to attempt to authenticate without entering a password, if the VNC Server supports this feature and all the necessary network infrastructure is in place.
For more information, see
vnc_Viewer_enableSingleSignOn()
.
-
public AnnotationManager
getAnnotationManager
() throws Library.VncException¶ Obtains the Viewer’s
AnnotationManager
for handling annotation operations.
-
public ConnectionHandler
getConnectionHandler
() throws Library.VncException¶ Returns the viewer’s
ConnectionHandler
for accepting connections.
-
public ConnectionStatus
getConnectionStatus
()¶ Returns the status of the viewer’s connection.
For more information, see
vnc_Viewer_getConnectionStatus()
.
-
public String
getDisconnectMessage
()¶ Returns a human-readable message sent by the server for the last disconnection, or
null
if the last disconnection was not initiated by the server.For more information, see
vnc_Viewer_getDisconnectMessage()
.
-
public String
getDisconnectReason
()¶ Returns a string ID representing the reason for the last viewer disconnection.
For more information, see
vnc_Viewer_getDisconnectReason()
.
-
public DisplayManager
getDisplayManager
() throws Library.VncException¶ Obtains the Viewer’s
DisplayManager
, for managing the list of displays made available by the Server to the Viewer.
-
public Encoding
getEncoding
()¶ Gets the currently set encoding, whether automatically determined or set by
Viewer.setEncoding()
.For more information, see
vnc_Viewer_getEncoding()
.
-
public EncryptionLevel
getEncryptionLevel
()¶ Returns the Viewer’s current encryption level.
For more information, see
vnc_Viewer_getEncryptionLevel()
.
-
public MessagingManager
getMessagingManager
() throws Library.VncException¶ Obtains the Viewer’s Messaging Manager for handling messaging
-
public String
getPeerAddress
() throws Library.VncException¶ Returns the address of the viewer’s server.
For more information, see
vnc_Viewer_getPeerAddress()
.
-
public PictureQuality
getPictureQuality
()¶ Returns the viewer’s current picture quality.
For more information, see
vnc_Viewer_getPictureQuality()
.
-
public byte[]
getServerPointerFbData
() throws Library.VncException¶ Gets the current mouse cursor image from the Server for rendering locally. The returned object is valid until the next call to getServerPointerFbData().
New in version 1.10.
-
public int
getServerPointerFbHeight
()¶ Gets the height of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbHeight()
.
-
public ImmutablePixelFormat
getServerPointerFbPixelFormat
() throws Library.VncException¶ Gets the pixel format of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbPixelFormat()
.
-
public int
getServerPointerFbWidth
()¶ Gets the width of the pointer framebuffer.
For more information, see
vnc_Viewer_getServerPointerFbWidth()
.
-
public int
getServerPointerXOffset
()¶ Gets the X offset of the “hotspot” of the pointer.
For more information, see
vnc_Viewer_getServerPointerXOffset()
.
-
public int
getServerPointerYOffset
()¶ Gets the Y offset of the “hotspot” of the pointer.
For more information, see
vnc_Viewer_getServerPointerYOffset()
.
-
public byte[]
getViewerFbData
(int x, int y, int w, int h) throws Library.VncException¶ Returns the viewer framebuffer data for the given rectangle. This method is rarely useful, and is inefficient. Typically, efficient access to the framebuffer is implemented either by:
- using the
setViewerFb
method to pass ajava.nio.ByteBuffer
to the SDK, allowing the pixels to be accessed using theByteBuffer
‘s methods; - or, on Android, using the
getViewerFbData(int,int,int,int,Object,int,int)
method to paint directly into an AndroidBitmap
.
- using the
-
public void
getViewerFbData
(int x, int y, int w, int h, Object bitmap, int targetX, int targetY) throws Library.VncException¶ Draw the framebuffer data into an Android
Bitmap
object. This method is only supported on Android. It provides improved performance over the standard methods provided by theBitmap
class, which are able to reinitialise the bitmap from aByteBuffer
, but do not provide efficient updating of subregions of the bitmap.The rectangle described by
(x, y, w, h)
describes a region in the Viewer’s framebuffer which is to be painted into the Android Bitmap with the top-left of the rectangle at coordinates(targetX, targetY)
.The Bitmap must use either the
ARGB_8888
orRGB_565
pixel formats, and the Viewer’s framebuffer must have been previously configured using the SDK’s matching pixel format ofPixelFormat.bgr888
orPixelFormat.rgb565
respectively. In the case of ARGB data,getViewerFbData
does not fill in the alpha channel, soBitmap.setHasAlpha(false)
must be called.Parameters: - bitmap – An
android.graphics.Bitmap
object.
See also: android.graphics.Bitmap documentation
- bitmap – An
-
public int
getViewerFbHeight
()¶ Gets the height of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbHeight()
.
-
public ImmutablePixelFormat
getViewerFbPixelFormat
() throws Library.VncException¶ Gets the pixel format of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbPixelFormat()
.
-
public int
getViewerFbStride
()¶ Returns the stride of the viewer framebuffer data in pixels, that is, the number of pixels from the start of each row until the start of the next.
For more information, see
vnc_Viewer_getViewerFbStride()
.
-
public int
getViewerFbWidth
()¶ Gets the width of the viewer framebuffer.
For more information, see
vnc_Viewer_getViewerFbWidth()
.
-
public void
releaseAllKeys
() throws Library.VncException¶ Send key up events for all currently pressed keys.
For more information, see
vnc_Viewer_releaseAllKeys()
.
-
public void
sendAuthenticationResponse
(boolean ok, String user, String passwd) throws Library.VncException¶ Provides the SDK with the result of a username/password request.
For more information, see
vnc_Viewer_sendAuthenticationResponse()
.
-
public void
sendClipboardText
(String text) throws Library.VncException¶ Copies the given text to the server’s clipboard.
For more information, see
vnc_Viewer_sendClipboardText()
.
-
public void
sendKeyDown
(int keysym, int keyCode) throws Library.VncException¶ Sends a key down (press) event to the server.
For more information, see
vnc_Viewer_sendKeyDown()
.
-
public void
sendKeyUp
(int keyCode) throws Library.VncException¶ Sends a key up (release) event to the server.
For more information, see
vnc_Viewer_sendKeyUp()
.
-
public void
sendPeerVerificationResponse
(boolean ok) throws Library.VncException¶ Provides the SDK with the response to the
Viewer.PeerVerificationCallback.verifyPeer()
request.For more information, see
vnc_Viewer_sendPeerVerificationResponse()
.
-
public void
sendPointerEvent
(int x, int y, java.lang.Iterable<MouseButton> buttonState, boolean rel) throws Library.VncException¶ Sends a pointer event to the server.
For more information, see
vnc_Viewer_sendPointerEvent()
.
-
public void
sendScrollEvent
(int delta, MouseWheel axis) throws Library.VncException¶ Sends a scroll wheel event to the server.
For more information, see
vnc_Viewer_sendScrollEvent()
.
-
public void
setAuthenticationCallback
(Viewer.AuthenticationCallback callback) throws Library.VncException¶ Sets the callback to be called when a username and/or password is required.
For more information, see
vnc_Viewer_setAuthenticationCallback()
.
-
public void
setConnectionCallback
(Viewer.ConnectionCallback callback) throws Library.VncException¶ Sets the callbacks for the Viewer to call when various events occur during its lifetime.
For more information, see
vnc_Viewer_setConnectionCallback()
.
-
public void
setEncoding
(Encoding encoding) throws Library.VncException¶ Enables the use of a specific encoding for the session.
For more information, see
vnc_Viewer_setEncoding()
.
-
public void
setEncryptionLevel
(EncryptionLevel level) throws Library.VncException¶ Sets the desired encryption level of the session from the range of options enumerated by
ENCRYPTION_LEVEL
.For more information, see
vnc_Viewer_setEncryptionLevel()
.
-
public void
setFramebufferCallback
(Viewer.FramebufferCallback callback) throws Library.VncException¶ Sets the framebuffer callback for this viewer.
For more information, see
vnc_Viewer_setFramebufferCallback()
.
-
public void
setPeerVerificationCallback
(Viewer.PeerVerificationCallback callback) throws Library.VncException¶ Sets the callbacks to be called to verify the identity of the peer (server).
For more information, see
vnc_Viewer_setPeerVerificationCallback()
.
-
public void
setPictureQuality
(PictureQuality quality) throws Library.VncException¶ Sets the desired picture quality of the session from the range of options enumerated by
PICTURE_QUALITY
.For more information, see
vnc_Viewer_setPictureQuality()
.
-
public void
setServerEventCallback
(Viewer.ServerEventCallback callback) throws Library.VncException¶ Sets the server event callback for this viewer.
For more information, see
vnc_Viewer_setServerEventCallback()
.
-
public void
setServerPointerCallback
(Viewer.ServerPointerCallback callback) throws Library.VncException¶ Sets the server pointer callback for this viewer.
For more information, see
vnc_Viewer_setServerPointerCallback()
.
-
public void
setServerPointerFbPixelFormat
(ImmutablePixelFormat pf)¶ Sets the pixel format of the pointer framebuffer.
For more information, see
vnc_Viewer_setServerPointerFbPixelFormat()
.
-
public void
setServerPointerPosPeriod
(int periodMs)¶ Sets the rate with which serverPointerPos callback will be called.
For more information, see
vnc_Viewer_setServerPointerPosPeriod()
.
-
public void
setViewerFb
(java.nio.ByteBuffer pixels, ImmutablePixelFormat pf, int width, int height, int stride) throws Library.VncException¶ Sets the viewer framebuffer.
For more information, see
vnc_Viewer_setViewerFb()
.