Learn how to evaluate and integrate the VNC SDK
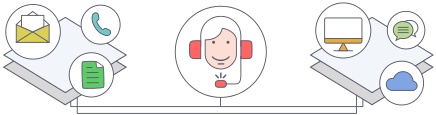
EventLoopWin.h¶
Implement a custom Windows HANDLE-based event loop. (more...)
Data structures
Modifier and Type | Name and Description |
---|---|
struct |
Callback receiving notifications for a Windows HANDLE-based event loop. |
Functions
Modifier and Type | Name and Description |
---|---|
function vnc_status_t | vnc_EventLoopWin_setCallback(const vnc_EventLoopWin_Callback *callback, void *userData) Sets the event loop callback. |
function int | vnc_EventLoopWin_getEvents(HANDLE *events) Gets the array of events that the SDK currently wishes to be notified of. |
function int | vnc_EventLoopWin_handleEvent(HANDLE event) Handles the given event (if any) and process expired timers. |
Detailed description
Implement a custom Windows HANDLE-based event loop.
The following functions are used for integrating the VNC SDK into an existing Windows HANDLE-based event loop. This could either be an application’s event loop implemented directly using MsgWaitForMultipleObjects
, or a third party event loop where the SDK’s event HANDLEs can be registered (for example using Qt’s QWinEventNotifier).
The functions in this file are all only available on Windows, and an assertion is used to verify that vnc_init() was used to create a Windows event loop.
See the basicViewerWin sample for an example of how to integrate this into an existing Windows event loop using WaitForMultipleObjects
.
To integrate into a third-party event loop (for use with a framework such as Qt), implement the vnc_EventLoopWin_Callback::eventUpdated and vnc_EventLoopWin_Callback::timerUpdated callbacks to create, update or destroy the appropriate notifiers for the framework. On receiving notifications, call vnc_EventLoopWin_handleEvent().
Functions
-
vnc_status_t
vnc_EventLoopWin_setCallback
(const vnc_EventLoopWin_Callback *callback, void *userData)¶ Sets the event loop callback.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Parameters
callback
-The new event loop callback.
- Return Value
InvalidArgument
-The vnc_EventLoopWin_Callback::eventUpdated callback is NULL
-
int
vnc_EventLoopWin_getEvents
(HANDLE *events)¶ Gets the array of events that the SDK currently wishes to be notified of.
- Return
- The number of events set in the output array
- Parameters
events
-An output array which will be filled with the list of events the SDK is using. The array pointed to by
events
must beMAXIMUM_WAIT_OBJECTS
in size.
-
int
vnc_EventLoopWin_handleEvent
(HANDLE event)¶ Handles the given event (if any) and process expired timers.
This should be called whenever a timer expires or an event occurs. If a timer expires and no event has been signalled, pass NULL as the handle to process timer events only.
- Return
- The number of milliseconds until the next timer expires
- Parameters
event
-The event which has been signalled.
-
struct
vnc_EventLoopWin_Callback
¶ - #include <EventLoopWin.h>
Callback receiving notifications for a Windows HANDLE-based event loop.
Public Members
-
void(* vnc_EventLoopWin_Callback::eventUpdated) (void *userData, HANDLE event, vnc_bool_t add)
Notification that a Windows event is being added or removed.
This callback is required.
The implementation should call vnc_EventLoopWin_handleEvent() whenever a HANDLE owned by the SDK is signalled. This callback does not get called for events that were created by the SDK prior to the callback being set; the implementation should use vnc_EventLoopWin_getEvents() to query those when vnc_EventLoopWin_setCallback() is called.
- Parameters
-
void(* vnc_EventLoopWin_Callback::timerUpdated) (void *userData, int expiryMs)
Notification that the timer expiry period has been updated.
When the specified expiry time has passed, the implementation should call vnc_EventLoopWin_handleEvent().
This callback is optional, and is only needed if integrating with a third-party event loop rather than calling vnc_EventLoopWin_handleEvent() directly to a create a custom blocking event loop.
- Parameters
expiryMs
-The maximum time that should be allowed to pass before the next call to vnc_EventLoopWin_handleEvent(), in milliseconds
-