Learn how to evaluate and integrate the VNC SDK
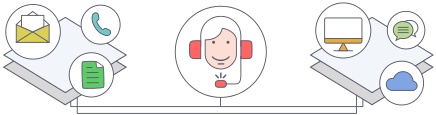
DirectTcp.h¶
Listens for or establishes connections using Direct TCP. (more...)
Data structures
Modifier and Type | Name and Description |
---|---|
struct | vnc_DirectTcpListener_Callback Callback for filtering incoming TCP connections. |
Defines
Modifier and Type | Name and Description |
---|---|
#define |
The default port for VNC direct TCP connections. |
Typedefs
Modifier and Type | Name and Description |
---|---|
typedef struct vnc_DirectTcpListener |
Listener used to receive incoming TCP connections. |
typedef struct vnc_DirectTcpConnector |
Connector used to make outgoing TCP connections. |
Functions
Modifier and Type | Name and Description |
---|---|
function vnc_DirectTcpListener * | vnc_DirectTcpListener_create(int port, const char *addressList, vnc_ConnectionHandler *connectionHandler, const vnc_DirectTcpListener_Callback *callback, void *userData) Begin listening for incoming TCP connections on the given port (IPv4 and IPv6). |
function void | vnc_DirectTcpListener_destroy(vnc_DirectTcpListener *listener) Destroys the TCP listener. |
function vnc_DirectTcpConnector * | vnc_DirectTcpConnector_create() Creates a new TCP Connector which is used to make outgoing connections to TCP listeners. |
function vnc_status_t | vnc_DirectTcpConnector_setProxySettings(vnc_DirectTcpConnector *connector, vnc_bool_t systemProxy, const char *proxyUrl) Set proxy server settings for this TCP Connector. |
function void | vnc_DirectTcpConnector_destroy(vnc_DirectTcpConnector *connector) Destroys the TCP Connector. |
function vnc_status_t | vnc_DirectTcpConnector_connect(vnc_DirectTcpConnector *connector, const char *hostOrIpAddress, int port, vnc_ConnectionHandler *connectionHandler) Begins an outgoing TCP connection to the given hostname or IP address. |
Detailed description
Listens for or establishes connections using Direct TCP.
- Since
- 1.2
Defines
-
VNC_DIRECT_TCP_DEFAULT_PORT
¶ The default port for VNC direct TCP connections.
Typedefs
-
typedef struct vnc_DirectTcpListener
vnc_DirectTcpListener
¶ Listener used to receive incoming TCP connections.
-
typedef struct vnc_DirectTcpConnector
vnc_DirectTcpConnector
¶ Connector used to make outgoing TCP connections.
Functions
-
vnc_DirectTcpListener *
vnc_DirectTcpListener_create
(int port, const char *addressList, vnc_ConnectionHandler *connectionHandler, const vnc_DirectTcpListener_Callback *callback, void *userData)¶ Begin listening for incoming TCP connections on the given port (IPv4 and IPv6).
To stop listening, destroy the TCP listener.
- Return
- Returns a new TCP listener on success, or NULL in the case of an error, in which case vnc_getLastError() can be used to get the error code.
- Since
- 1.2
- Parameters
port
-The port number to listen on.
addressList
-A comma-separated list of addresses to listen on. If empty or null then listening starts on all addresses.
connectionHandler
-The object which shall handle incoming connections (if not rejected by the filter function). It is either a server or a viewer. It must be destroyed after the TCP listener.
callback
-An optional callback to filter incoming connections.
userData
-An optional pointer to user data.
- Return Value
AddressError
-There was an error listening on the specified address
NotEnabled
-The SDK does not have the Direct TCP add-on enabled
-
void
vnc_DirectTcpListener_destroy
(vnc_DirectTcpListener *listener)¶ Destroys the TCP listener.
Any ongoing connections are not affected, but new connections will not be accepted.
- Since
- 1.2
- Parameters
listener
-The TCP listener to destroy.
-
vnc_DirectTcpConnector *
vnc_DirectTcpConnector_create
(void)¶ Creates a new TCP Connector which is used to make outgoing connections to TCP listeners.
- Return
- Returns a new TCP Connector on success, or NULL in the case of an error, in which case vnc_getLastError() can be used to get the error code.
- Since
- 1.2
- Return Value
NotEnabled
-The SDK does not have the Direct TCP add-on enabled
-
vnc_status_t
vnc_DirectTcpConnector_setProxySettings
(vnc_DirectTcpConnector *connector, vnc_bool_t systemProxy, const char *proxyUrl)¶ Set proxy server settings for this TCP Connector.
The settings are adopted for all subsequent outgoing connections for this connector. The proxy settings can be either the system configured settings or as specified in a supplied settings string. If the proxy server requires authentication then full user credentials (i.e. username and password) must be configured. Any connection error arising from the proxy settings is reported when an outgoing connection is attempted, see vnc_DirectTcpConnector_connect
- Return
- vnc_success is returned on success or vnc_failure in the case of an error, in which case vnc_getLastError() can be used to get the error code.
- Since
- 1.2
- Parameters
connector
-The TCP connector that will employ the proxy settings
systemProxy
-Set this to vnc_true to employ the currently configured system proxy settings. When set to vnc_false,
proxyUrl
is used to specify the proxy settings.proxyUrl
-A string specifying a proxy server, plus user credentials where these are required by an authenticated proxy server. The format of the string is: type://username:password@address::port Type is either ‘socks’ or ‘http’ Example: socks://fred:X$fr£4Pw@mySocks5Proxy.here.com::8888 If
systemProxy
is vnc_false and the proxyUrl string is null or empty then this is treated as a request to stop using a proxy server.
- Return Value
InvalidArgument
-proxyUrl
does not conform to the required format (see above)
-
void
vnc_DirectTcpConnector_destroy
(vnc_DirectTcpConnector *connector)¶ Destroys the TCP Connector.
- Since
- 1.2
- Parameters
connector
-The TCP connector to destroy.
-
vnc_status_t
vnc_DirectTcpConnector_connect
(vnc_DirectTcpConnector *connector, const char *hostOrIpAddress, int port, vnc_ConnectionHandler *connectionHandler)¶ Begins an outgoing TCP connection to the given hostname or IP address.
The connection will be handled by the supplied
connectionHandler
.The connection shall be via direct TCP unless a preceding call was made to vnc_DirectTcpConnector_setProxySettings to result in connections being made via a proxy server.
Connection errors are notified using the server or viewer’s callbacks (depending on the type of the connection handler used). This includes errors arising when resolving a specified hostname.
- Return
- vnc_success is returned on success or vnc_failure in the case of an error, in which case vnc_getLastError() can be used to get the error code.
- Since
- 1.2
- Parameters
connector
-The TCP connector that is to begin the connection.
hostOrIpAddress
-The DNS hostname or IP address to connect to.
port
-The port number to connect to. Note: The default port for VNC connections can be obtained using VNC_DIRECT_TCP_DEFAULT_PORT
connectionHandler
-The object (a Viewer or a Server) to handle the connection.
- Return Value
InvalidArgument
-port
is invalid, orconnectionHandler
is a null pointer
-
struct
vnc_DirectTcpListener_Callback
¶ - #include <DirectTcp.h>
Callback for filtering incoming TCP connections.
Public Members
-
vnc_bool_t(* vnc_DirectTcpListener_Callback::filterConnection) (void *userData, vnc_DirectTcpListener *listener, const char *ipAddress, int port)
Notification to provide address-based filtering of incoming connections.
This callback is optional.
- Return
- vnc_true to allow the connection, or vnc_false to deny, in which case the connection will be closed.
- Since
- 1.2
- Parameters
listener
-The listening object that performed this callback.
ipAddress
-The IP address of the remote end that is attempting to make a connection. The address is presented in a human-readable form such as 122.16.224.1 (IPv4) and 2001:dc8:0:1534:0:867:6:1 (IPv6)
port
-The port on which a connection is being attempted.
-