Learn how to evaluate and integrate the VNC SDK
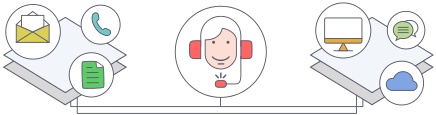
DataStore.h¶
Creates a persistent data store. (more...)
Data structures
Modifier and Type | Name and Description |
---|---|
struct |
Callback providing custom storage of data used by the SDK. |
Functions
Modifier and Type | Name and Description |
---|---|
function vnc_status_t | vnc_DataStore_createCustomStore(const vnc_DataStore_Callback *callback, void *userData) Creates a custom data store. |
function vnc_status_t | vnc_DataStore_createFileStore(const char *path) Creates a file data store. |
function vnc_status_t | vnc_DataStore_createRegistryStore(const char *registryPath) Creates a registry data store. |
function void | vnc_DataStore_createBrowserStore(const char *prefix) Creates a web browser data store, in DOM |
function void |
Destroys the current data store. |
Detailed description
Creates a persistent data store.
For both a Viewer and a Server, you must create a data store in which the SDK can store an automatically-generated RSA public/private key pair and retrieve it on demand. The RSA key is required for encryption and identity verification, and connections cannot be established without it. Note the SDK also stores other data, for example VNC Cloud discovery information.
You can either call an appropriate method to let the SDK create and manage a suitable data store for you, or else call vnc_DataStore_createCustomStore() to create your own store in which you put and get data when notified by the SDK. Note that key generation itself occurs at the time of the first connection unless you force it by calling vnc_RsaKey_getDetails() after you create your data store (this might help prevent connection failures on slower platforms such as the Raspberry Pi).
Only one data store can exist in your Viewer or Server at a time. If you create a new data store, an existing data store is automatically replaced. Any SDK functions that read or write to the data store will produce a DataStoreError
while no data store exists.
Note that functions declared in this header may be called before calling vnc_init().
Functions
-
vnc_status_t
vnc_DataStore_createCustomStore
(const vnc_DataStore_Callback *callback, void *userData)¶ Creates a custom data store.
You are responsible for storing and retrieving data in the correct format when notified by the SDK.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Parameters
callback
-A vnc_DataStore_Callback structure.
userData
-Custom data to pass through to callback function implementations.
- Return Value
InvalidArgument
-One or more mandatory callbacks in
callback
isNULL
.
-
vnc_status_t
vnc_DataStore_createFileStore
(const char *path)¶ Creates a file data store.
The SDK manages storing and retrieving data for you. Note this type of store is not available on the HTML5 platform.
Note that the data store contains sensitive information such as the RSA private key, and therefore it must not be readable by any potential attacker on the system. For this reason it is essential that the parent directory/folder of the given path has been created with appropriate permissions which do not allow any access to other users on the system.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Parameters
path
-A full path to and file name for the data store. You can specify any file extension; a binary file will be created.
- Return Value
InvalidArgument
-path
is invalid.DataStoreError
-The file could not be opened or created.
-
vnc_status_t
vnc_DataStore_createRegistryStore
(const char *registryPath)¶ Creates a registry data store.
The SDK manages storing and retrieving data for you. Note this type of store is only available on Windows.
Note that the data store contains sensitive information such as the RSA private key, and therefore it must not be readable by any potential attacker on the system. For this reason it is essential that the registry key have appropriate permissions which do not allow any access to other users on the system.
- Return
- vnc_success or vnc_failure, in which case call vnc_getLastError() to get the error code.
- Parameters
registryPath
-A suitable path to and name for a registry key, for example
HKEY_LOCAL_USER\SOFTWARE\Company\Application\MyKey
.
- Return Value
InvalidArgument
-registryPath
is invalid.DataStoreError
-The registry key could not be opened.
-
void
vnc_DataStore_createBrowserStore
(const char *prefix)¶ Creates a web browser data store, in DOM
localStorage
.The SDK manages storing and retrieving data for you. Note this type of store is only available on the HTML5 platform.
- Parameters
prefix
-A prefix prepending to the
localStorage
key names, so that the SDK’s keys do not conflict with other libraries.
-
void
vnc_DataStore_destroyStore
(void)¶ Destroys the current data store.
-
struct
vnc_DataStore_Callback
¶ - #include <DataStore.h>
Callback providing custom storage of data used by the SDK.
Public Members
-
void(* vnc_DataStore_Callback::put) (void *userData, const char *key, const vnc_DataBuffer *value)
Requests storing data in your custom data store.
This callback is required for a custom data store.
In your implementation, associate the data contained in
value
with the given key. If a value with the same key exists already, overwrite it. Ifvalue
is NULL, remove any existing value stored for the key. Note the vnc_DataBuffer is destroyed when the callback function returns, so copy data out of the buffer rather than storing the buffer itself.- Parameters
key
-A NULL-terminated string.
value
-A vnc_DataBuffer to store, or NULL to remove an existing value with the same key from your custom data store.
-
vnc_DataBuffer *(* vnc_DataStore_Callback::get) (void *userData, const char *key)
Requests data stored in your custom data store.
This callback is required for a custom data store.
In your implementation, you should retrieve the value associated with
key
and return it as a vnc_DataBuffer, or else NULL if the key could not be found.- Return
- A vnc_DataBuffer.
- Parameters
key
-A NULL-terminated string.
-